Apply NMS on GraspsΒΆ
Get a GraspNet instance.
# GraspNetAPI example for grasp nms.
# change the graspnet_root path
####################################################################
graspnet_root = '/home/gmh/graspnet' # ROOT PATH FOR GRASPNET
####################################################################
sceneId = 1
annId = 3
from graspnetAPI import GraspNet
import open3d as o3d
import cv2
# initialize a GraspNet instance
g = GraspNet(graspnet_root, camera='kinect', split='train')
Loading and visualizing grasp lables before NMS.
# load grasps of scene 1 with annotation id = 3, camera = kinect and fric_coef_thresh = 0.2
_6d_grasp = g.loadGrasp(sceneId = sceneId, annId = annId, format = '6d', camera = 'kinect', fric_coef_thresh = 0.2)
print('6d grasp:\n{}'.format(_6d_grasp))
# visualize the grasps using open3d
geometries = []
geometries.append(g.loadScenePointCloud(sceneId = sceneId, annId = annId, camera = 'kinect'))
geometries += _6d_grasp.random_sample(numGrasp = 1000).to_open3d_geometry_list()
o3d.visualization.draw_geometries(geometries)
6d grasp:
----------
Grasp Group, Number=90332:
Grasp: score:0.9000000357627869, width:0.11247877031564713, height:0.019999999552965164, depth:0.029999999329447746, translation:[-0.09166837 -0.16910084 0.39480919]
rotation:
[[-0.81045675 -0.57493848 0.11227506]
[ 0.49874267 -0.77775514 -0.38256073]
[ 0.30727136 -0.25405255 0.91708326]]
object id:66
Grasp: score:0.9000000357627869, width:0.10030215978622437, height:0.019999999552965164, depth:0.019999999552965164, translation:[-0.09166837 -0.16910084 0.39480919]
rotation:
[[-0.73440629 -0.67870212 0.0033038 ]
[ 0.64608938 -0.70059127 -0.3028869 ]
[ 0.20788456 -0.22030747 0.95302087]]
object id:66
Grasp: score:0.9000000357627869, width:0.08487851172685623, height:0.019999999552965164, depth:0.019999999552965164, translation:[-0.10412319 -0.13797761 0.38312319]
rotation:
[[ 0.03316294 0.78667939 -0.61647028]
[-0.47164679 0.55612743 0.68430364]
[ 0.88116372 0.26806271 0.38947764]]
object id:66
......
Grasp: score:0.9000000357627869, width:0.11909123510122299, height:0.019999999552965164, depth:0.019999999552965164, translation:[-0.05140382 0.11790846 0.48782501]
rotation:
[[-0.71453273 0.63476181 -0.2941435 ]
[-0.07400083 0.3495101 0.93400562]
[ 0.69567728 0.68914449 -0.20276351]]
object id:14
Grasp: score:0.9000000357627869, width:0.10943549126386642, height:0.019999999552965164, depth:0.019999999552965164, translation:[-0.05140382 0.11790846 0.48782501]
rotation:
[[ 0.08162415 0.4604325 -0.88393396]
[-0.52200603 0.77526748 0.3556262 ]
[ 0.84902728 0.4323912 0.30362913]]
object id:14
Grasp: score:0.9000000357627869, width:0.11654743552207947, height:0.019999999552965164, depth:0.009999999776482582, translation:[-0.05140382 0.11790846 0.48782501]
rotation:
[[-0.18380146 0.39686993 -0.89928377]
[-0.61254776 0.66926688 0.42055583]
[ 0.76876676 0.62815309 0.12008961]]
object id:14
------------
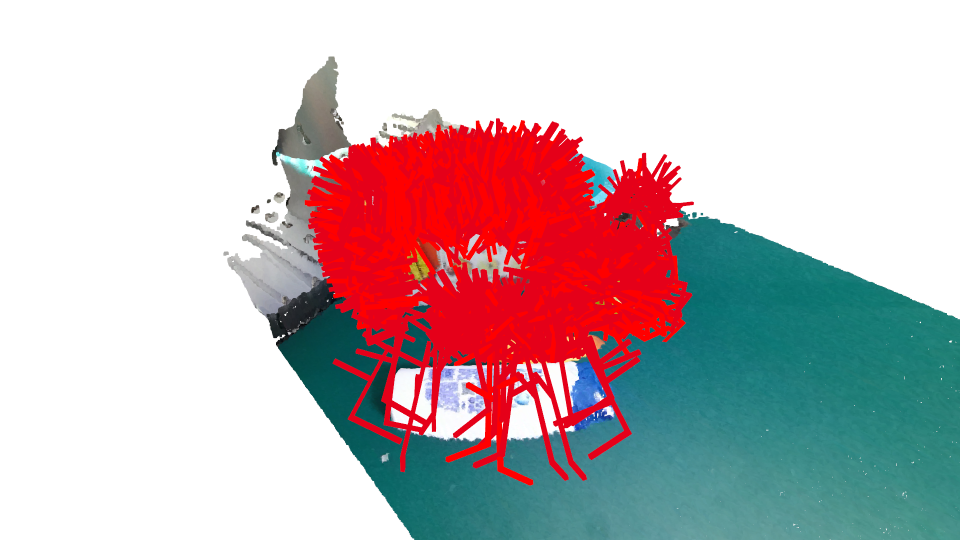
Apply nms to GraspGroup and visualizing the result.
nms_grasp = _6d_grasp.nms(translation_thresh = 0.1, rotation_thresh = 30 / 180.0 * 3.1416)
print('grasp after nms:\n{}'.format(nms_grasp))
# visualize the grasps using open3d
geometries = []
geometries.append(g.loadScenePointCloud(sceneId = sceneId, annId = annId, camera = 'kinect'))
geometries += nms_grasp.to_open3d_geometry_list()
o3d.visualization.draw_geometries(geometries)
grasp after nms:
----------
Grasp Group, Number=358:
Grasp: score:1.0, width:0.11948642134666443, height:0.019999999552965164, depth:0.03999999910593033, translation:[-0.00363996 0.03692623 0.3311775 ]
rotation:
[[ 0.32641056 -0.8457799 0.42203382]
[-0.68102902 -0.52005678 -0.51550031]
[ 0.65548128 -0.11915252 -0.74575269]]
object id:0
Grasp: score:1.0, width:0.12185929715633392, height:0.019999999552965164, depth:0.009999999776482582, translation:[-0.03486454 0.08384828 0.35117128]
rotation:
[[-0.00487804 -0.8475557 0.53068405]
[-0.27290785 -0.50941664 -0.81609803]
[ 0.96202785 -0.14880882 -0.22881967]]
object id:0
Grasp: score:1.0, width:0.04842342436313629, height:0.019999999552965164, depth:0.019999999552965164, translation:[0.10816982 0.10254505 0.50272578]
rotation:
[[-0.98109186 -0.01696888 -0.19279723]
[-0.1817532 0.42313483 0.88765001]
[ 0.06651681 0.90590769 -0.41821831]]
object id:20
......
Grasp: score:0.9000000357627869, width:0.006192661356180906, height:0.019999999552965164, depth:0.009999999776482582, translation:[0.0122985 0.29616502 0.53319722]
rotation:
[[-0.26423979 0.39734706 0.87880182]
[-0.95826042 -0.00504095 -0.28585231]
[-0.10915259 -0.91765451 0.38209397]]
object id:46
Grasp: score:0.9000000357627869, width:0.024634981527924538, height:0.019999999552965164, depth:0.009999999776482582, translation:[0.11430283 0.18761221 0.51991153]
rotation:
[[-0.17379239 -0.96953499 0.17262182]
[-0.9434278 0.11365268 -0.31149188]
[ 0.28238329 -0.2169912 -0.93443805]]
object id:70
Grasp: score:0.9000000357627869, width:0.03459500893950462, height:0.019999999552965164, depth:0.009999999776482582, translation:[0.02079188 0.11184558 0.50796509]
rotation:
[[ 0.38108557 -0.27480939 0.88275337]
[-0.92043257 -0.20266907 0.33425891]
[ 0.08704928 -0.93989623 -0.33017775]]
object id:20
----------
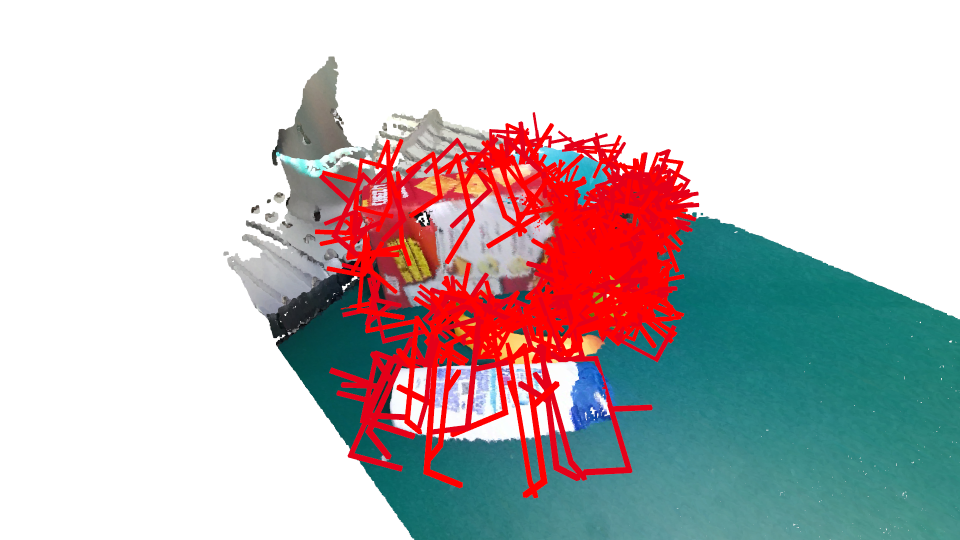